# Web infra
# CORS
Cross-origin resource sharing.
Client side
front page (browser): You control the resources allowed to be loaded with theCSP
header.resource
side (other website) : You control theclient side
(i.e. original page) with theAccess-Control-Allow-Origin
header.
# Header
# Connection
The Connection general header controls whether or not the network connection stays open after the current transaction finishes. If the value sent is keep-alive, the connection is persistent and not closed, allowing for subsequent requests to the same server to be done. Typically this choses if tcp session
is kept or not.
Connection: keep-alive
Connection: close
2
# X-Forwarded-For
The X-Forwarded-For (XFF) header is a de-facto standard header for identifying the originating IP address of a client connecting to a web server through an HTTP proxy or a load balancer.
When traffic is intercepted between clients and servers, server access logs contain the IP address of the proxy or load balancer only.
To see the original IP address of the client, the X-Forwarded-For request header is used.
This header is used for debugging, statistics, and generating location-dependent content and by design it exposes privacy sensitive information, such as the IP address of the client. Therefore the user's privacy must be kept in mind when deploying this header.
X-Forwarded-For: <client>, <proxy1>, <proxy2>
X-Forwarded-For: 2001:db8:85a3:8d3:1319:8a2e:370:7348
X-Forwarded-For: 203.0.113.195
X-Forwarded-For: 203.0.113.195, 70.41.3.18, 150.172.238.178
2
3
4
5
6
7
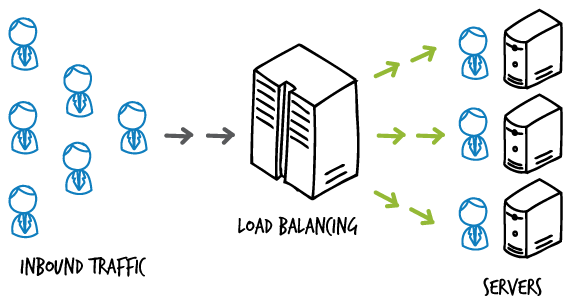
# Access-Control-Allow-Origin
MDN docopen in new window
Test a CORS.
curl -vk https://api.example.com -H 'Origin: your.origin.example.com'
# Content-Security-Policy
# script-src
# Referrer-Policy
A good articleopen in new window
# Permissions-Policy
A good articleopen in new window
# Curl
for target in '10.10.10.10' 'youtube.com'
do
curl -sSL "$target/api" \
-d 'url=https://mattrunks.com/en' \
-H 'Accept:application/json' \
-H 'Host: youtube.com' \
-o /dev/null \
-w "$target --> %{http_code}\n" \
-X POST
done
2
3
4
5
6
7
8
9
10
# Open Graph protocol
The Open Graph protocol enables any web page to become a rich object in a social graph. For instance, this is used on Facebook to allow any web page to have the same functionality as any other object on Facebook.
While many different technologies and schemas exist and could be combined together, there isn't a single technology which provides enough information to richly represent any web page within the social graph. The Open Graph protocol builds on these existing technologies and gives developers one thing to implement. Developer simplicity is a key goal of the Open Graph protocol which has informed many of the technical design decisions.
# Basic Metadata
To turn your web pages into graph objects, you need to add basic metadata to your page. We've based the initial version of the protocol on RDFa which means that you'll place additional <meta>
tags in the <head>
of your web page. The four required properties for every page are:
og:title
- The title of your object as it should appear within the graph, e.g., "The Rock".og:type
- The type of your object, e.g., "video.movie". Depending on the type you specify, other properties may also be required.og:image
- An image URL which should represent your object within the graph.og:url
- The canonical URL of your object that will be used as its permanent ID in the graph, e.g., "http://www.imdb.com/title/tt0117500/".
As an example, the following is the Open Graph protocol markup for The Rock on IMDB:
<html prefix="og: http://ogp.me/ns#">
<head>
<title>The Rock (1996)</title>
<meta property="og:title" content="The Rock" />
<meta property="og:type" content="video.movie" />
<meta property="og:url" content="http://www.imdb.com/title/tt0117500/" />
<meta property="og:image" content="http://ia.media-imdb.com/images/rock.jpg" />
...
</head>
...
</html>
2
3
4
5
6
7
8
9
10
11
# Nginx
pronouced Engine X
Configuration pitfallsopen in new window
# Variables
HTTP variablesopen in new window
# virtual host
example redirect HTTP to HTTPS
server {
listen 80;
return 301 https://$host$request_uri;
}
2
3
4
Example of vhost (from jenkinsopen in new window)
upstream jenkins {
keepalive 32;
server 127.0.0.1:8080;
}
server {
listen 80;
server_name jenkins.example.com;
root /var/run/jenkins/war/;
access_log /var/log/nginx/jenkins/access.log;
error_log /var/log/nginx/jenkins/error.log;
ignore_invalid_headers off; #pass through headers from Jenkins which are considered invalid by Nginx server.
location ~ "^/static/[0-9a-fA-F]{8}\/(.*)quot; {
#rewrite all static files into requests to the root
#E.g /static/12345678/css/something.css will become /css/something.css
rewrite "^/static/[0-9a-fA-F]{8}\/(.*)" /$1 last;
}
location /userContent {
root /var/lib/jenkins/;
if (!-f $request_filename){
rewrite (.*) /$1 last;
break;
}
sendfile on;
}
location / {
sendfile off;
proxy_pass http://jenkins;
proxy_redirect default;
proxy_http_version 1.1;
proxy_set_header Host $host;
proxy_set_header X-Real-IP $remote_addr;
proxy_set_header X-Forwarded-For $proxy_add_x_forwarded_for;
proxy_set_header X-Forwarded-Proto $scheme;
proxy_max_temp_file_size 0;
#this is the maximum upload size
client_max_body_size 10m;
client_body_buffer_size 128k;
proxy_connect_timeout 90;
proxy_send_timeout 90;
proxy_read_timeout 90;
proxy_buffering off;
proxy_request_buffering off;
proxy_set_header Connection "";
}
}
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
# TLS
worker_processes auto;
http {
ssl_session_cache shared:SSL:10m;
ssl_session_timeout 10m;
server {
listen 443 ssl;
server_name www.example.com;
keepalive_timeout 70;
ssl_certificate www.example.com.crt;
ssl_certificate_key www.example.com.key;
ssl_protocols TLSv1 TLSv1.1 TLSv1.2;
ssl_ciphers HIGH:!aNULL:!MD5;
...
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
# Config generator
simple config : https://www.digitalocean.com/community/tools/nginx
much complexe example : https://www.digitalocean.com/community/tools/nginx#?0.non_www=false&0.cdn&0.php=false&0.wordpress&0.django&0.proxy&0.proxy_path=%2Fjenkins&0.proxy_pass=http:%2F%2F127.0.0.1:8080&0.access_log_domain&0.error_log_domain
# Apache
Validate config before reload/restart
apachectl configtest
TLS config
...
SSLCertificateFile "/etc/ssl/certs/www.example.com.cert"
SSLCertificateKeyFile "/etc/ssl/private/www.example.com.key"
SSLCACertificateFile "/etc/ssl/certs/intermediate.ca.example.com.cert"
...
2
3
4
5
6